Database Tables Used
model_has_roles
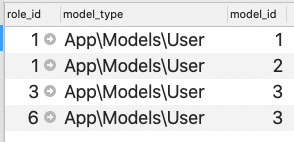
The role_id is foreign to roles table, straight-forward. model_type is self-explanatory. model_id is the id for the users table.
Query based on roles
Please note the ->hasRoles does not work with query. That works on the user model as a method to check something.
For query perhaps we can use the following:
1 | $query->role($userRole); |
How to get all the roles of the users? Further putting all the role ids into an Array.
1 2 3 4 5 6 7 8 9 10 11 12 | $userRoleIdsArray = []; $userRoles = User::whereId(Auth::id())->with('roles')->first(); if (!empty($userRoles)) { foreach ($userRoles->roles as $role) { array_push($userRoleIdsArray, $role->id); } unset($userRoles); } else { Log::error("Plan.userRow not found for the user Id -" . Auth::id()); } |
More details can be found here.
How to write a relationship to Roles Model since we do not have one by default?
This is useful when let’s say we want to have a plans table and we want plans and roles to be mapped with a pivot table. So we can create a many-to-many relationship between roles and plans. Here’s how to do it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Role extends \Spatie\Permission\Models\Role { use HasFactory; public function plans() { return $this->belongsToMany(Plan::class); } } |
Read more about this over here.